Android Fragment Lifecycle Explanation
A Fragment in Android is a reusable portion of the UI associated with an Activity. It has its own lifecycle that closely integrates with the Activity’s lifecycle. This allows fragments to manage their UI and behavior efficiently.
Fragment Lifecycle Callbacks
-
onAttach(Context context):
- Called when the fragment is associated with an Activity.
- Provides the Context of the host Activity.
- Perform operations that require the Activity context.
-
onCreate(Bundle savedInstanceState):
- Triggered when the fragment is first created.
- Initialize non-UI components like variables, ViewModels, or data structures.
- Similar to an Activity’s
onCreate()
but does not inflate the layout.
-
onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState):
- Called to inflate the fragment’s UI layout.
- Return the root
View
of the fragment’s layout.
Example:
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState:Bundle?): View? { return inflater.inflate(R.layout.fragment_example, container, false) }
-
onViewCreated(View view, Bundle savedInstanceState):
- Called immediately after
onCreateView()
. - Perform view-related tasks such as setting up UI components, listeners, or observers.
- Called immediately after
-
onStart():
- Called when the fragment becomes visible to the user.
- The fragment enters the “Started” state.
- Typically used to start animations or other visual elements.
-
onResume():
- Triggered when the fragment is active and interactive (in the foreground).
- The fragment enters the “Resumed” state.
-
onPause():
- Called when the fragment is no longer in focus but still partially visible.
- Pause tasks like animations or media playback.
-
onStop():
- Called when the fragment is no longer visible to the user.
- Release resources or save the current state.
-
onDestroyView():
- Called when the fragment’s view is destroyed.
- Clean up resources related to the UI to avoid memory leaks.
-
onDestroy():
- Called when the fragment is destroyed.
- Perform final cleanup for the fragment’s state and components.
-
onDetach():
- Called when the fragment is detached from its host Activity.
- The fragment’s lifecycle is now complete.
Fragment Lifecycle Flow
-
Fragment Created:
- onAttach() → onCreate() → onCreateView() → onViewCreated() → onStart() → onResume()
- The fragment becomes visible and interactive.
-
Fragment Interrupted:
- onPause() → onStop()
- The fragment loses focus or visibility.
-
Fragment Destroyed:
- onDestroyView() → onDestroy() → onDetach()
- The fragment and its resources are cleaned up.
Visual Representation
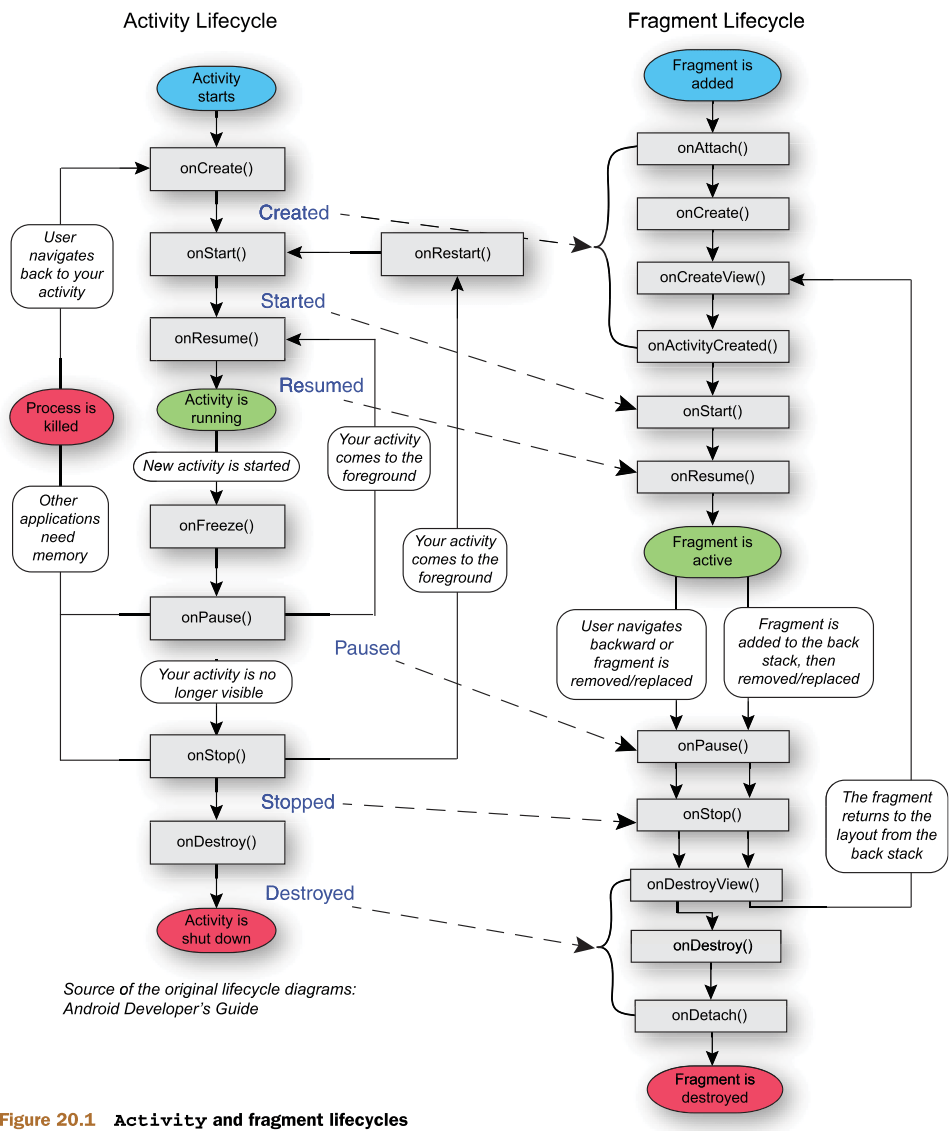
Key Notes
- onCreateView() is the key point where the fragment’s UI is inflated.
- Use onViewCreated() to handle UI setup like listeners or data binding.
- Always release resources like adapters or observers in onDestroyView() to prevent memory leaks.
- onAttach() and onDetach() connect and disconnect the fragment from its host Activity.
- Fragments are often used in dynamic and modular UI designs, especially with navigation components.